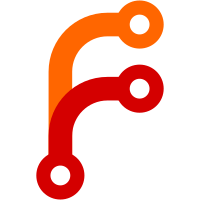
On DragonFly and NetBSD build fails with src/common/virtual_buffer.cpp src/common/virtual_buffer.cpp:16:10: fatal error: sys/sysinfo.h: No such file or directory #include <sys/sysinfo.h> ^~~~~~~~~~~~~~~
44 lines
867 B
C++
44 lines
867 B
C++
// Copyright 2020 yuzu Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#ifdef _WIN32
|
|
#include <windows.h>
|
|
#else
|
|
#include <sys/mman.h>
|
|
#endif
|
|
|
|
#include "common/assert.h"
|
|
#include "common/virtual_buffer.h"
|
|
|
|
namespace Common {
|
|
|
|
void* AllocateMemoryPages(std::size_t size) {
|
|
#ifdef _WIN32
|
|
void* base{VirtualAlloc(nullptr, size, MEM_COMMIT, PAGE_READWRITE)};
|
|
#else
|
|
void* base{mmap(nullptr, size, PROT_READ | PROT_WRITE, MAP_ANON | MAP_PRIVATE, -1, 0)};
|
|
|
|
if (base == MAP_FAILED) {
|
|
base = nullptr;
|
|
}
|
|
#endif
|
|
|
|
ASSERT(base);
|
|
|
|
return base;
|
|
}
|
|
|
|
void FreeMemoryPages(void* base, [[maybe_unused]] std::size_t size) {
|
|
if (!base) {
|
|
return;
|
|
}
|
|
#ifdef _WIN32
|
|
ASSERT(VirtualFree(base, 0, MEM_RELEASE));
|
|
#else
|
|
ASSERT(munmap(base, size) == 0);
|
|
#endif
|
|
}
|
|
|
|
} // namespace Common
|