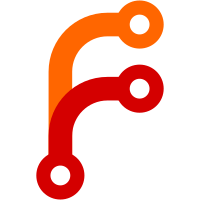
This formats all copyright comments according to SPDX formatting guidelines. Additionally, this resolves the remaining GPLv2 only licensed files by relicensing them to GPLv2.0-or-later.
32 lines
649 B
C++
32 lines
649 B
C++
// SPDX-FileCopyrightText: Copyright 2020 yuzu Emulator Project
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include <atomic>
|
|
#include <chrono>
|
|
|
|
namespace VideoCore {
|
|
class ShaderNotify {
|
|
public:
|
|
[[nodiscard]] int ShadersBuilding() noexcept;
|
|
|
|
void MarkShaderComplete() noexcept {
|
|
++num_complete;
|
|
}
|
|
|
|
void MarkShaderBuilding() noexcept {
|
|
++num_building;
|
|
}
|
|
|
|
private:
|
|
std::atomic_int num_building{};
|
|
std::atomic_int num_complete{};
|
|
int report_base{};
|
|
|
|
bool completed{};
|
|
int num_when_completed{};
|
|
std::chrono::steady_clock::time_point complete_time;
|
|
};
|
|
} // namespace VideoCore
|