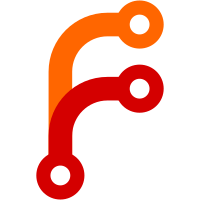
This removes explicit checks sprinkled all over the codebase to instead just have the SW rasterizer expose an implementation with no-ops for most operations.
49 lines
1.5 KiB
C++
49 lines
1.5 KiB
C++
// Copyright 2015 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include "common/common_types.h"
|
|
|
|
namespace Pica {
|
|
namespace Shader {
|
|
struct OutputVertex;
|
|
}
|
|
}
|
|
|
|
namespace VideoCore {
|
|
|
|
class RasterizerInterface {
|
|
public:
|
|
virtual ~RasterizerInterface() {}
|
|
|
|
/// Initialize API-specific GPU objects
|
|
virtual void InitObjects() = 0;
|
|
|
|
/// Reset the rasterizer, such as flushing all caches and updating all state
|
|
virtual void Reset() = 0;
|
|
|
|
/// Queues the primitive formed by the given vertices for rendering
|
|
virtual void AddTriangle(const Pica::Shader::OutputVertex& v0,
|
|
const Pica::Shader::OutputVertex& v1,
|
|
const Pica::Shader::OutputVertex& v2) = 0;
|
|
|
|
/// Draw the current batch of triangles
|
|
virtual void DrawTriangles() = 0;
|
|
|
|
/// Commit the rasterizer's framebuffer contents immediately to the current 3DS memory framebuffer
|
|
virtual void FlushFramebuffer() = 0;
|
|
|
|
/// Notify rasterizer that the specified PICA register has been changed
|
|
virtual void NotifyPicaRegisterChanged(u32 id) = 0;
|
|
|
|
/// Notify rasterizer that any caches of the specified region should be flushed to 3DS memory.
|
|
virtual void FlushRegion(PAddr addr, u32 size) = 0;
|
|
|
|
/// Notify rasterizer that any caches of the specified region should be discraded and reloaded from 3DS memory.
|
|
virtual void InvalidateRegion(PAddr addr, u32 size) = 0;
|
|
};
|
|
|
|
}
|